5 Best PHP Libraries to Create Charts and Graphs
No matter what industry you work in, data visualization is an important part of conveying information. When it comes to programming, there are a number of ways to create charts and graphs. In this blog post, we will take a look at five of the best PHP libraries for creating charts.
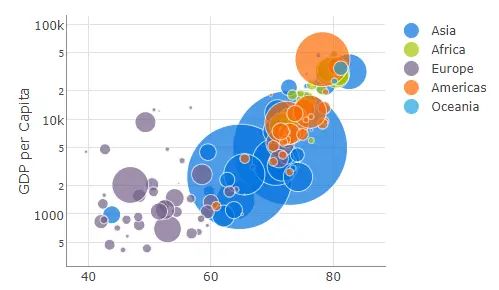
pChart
pChart is an open-source PHP library that is ideal for creating a wide range of chart types, including line graphs, pie charts, bar charts, and radar graphs.
With pChart, you can easily create anti-aliased charts or pictures from your web server in just minutes; display the result in any client browser, send it by mail, or even insert it into PDF documents!
In order to get started, however, you must make sure the GD and FreeType PHP extensions are properly installed on your server so that pChart can do its job correctly. pChart also has the ability to create dynamic images by using the GD2 image processing library. This makes it a great choice for creating charts that need to be updated frequently.
/* CAT:Pie charts */ /* pChart library inclusions */ include(__DIR__ . "/../library/pData.php"); include(__DIR__ . "/../library/pDraw.php"); include(__DIR__ . "/../library/pPie.php"); include(__DIR__ . "/../library/pImage.php"); /* Create and populate the pData object */ $MyData = new pData(); $MyData->addPoints(array(40,60,15,10,6,4),"ScoreA"); $MyData->setSerieDescription("ScoreA","Application A"); /* Define the absissa serie */ $MyData->addPoints(array("<10","10<>20","20<>40","40<>60","60<>80",">80"),"Labels"); $MyData->setAbscissa("Labels"); /* Create the pChart object */ $myPicture = new pImage(700,230,$MyData); /* Draw a solid background */ $Settings = array("R"=>173, "G"=>152, "B"=>217, "Dash"=>1, "DashR"=>193, "DashG"=>172, "DashB"=>237); $myPicture->drawFilledRectangle(0,0,700,230,$Settings); /* Draw a gradient overlay */ $Settings = array("StartR"=>209, "StartG"=>150, "StartB"=>231, "EndR"=>111, "EndG"=>3, "EndB"=>138, "Alpha"=>50); $myPicture->drawGradientArea(0,0,700,230,DIRECTION_VERTICAL,$Settings); $myPicture->drawGradientArea(0,0,700,20,DIRECTION_VERTICAL,array("StartR"=>0,"StartG"=>0,"StartB"=>0,"EndR"=>50,"EndG"=>50,"EndB"=>50,"Alpha"=>100)); /* Add a border to the picture */ $myPicture->drawRectangle(0,0,699,229,array("R"=>0,"G"=>0,"B"=>0)); /* Write the picture title */ $myPicture->setFontProperties(array("FontName"=>"../fonts/Silkscreen.ttf","FontSize"=>6)); $myPicture->drawText(10,13,"pPie - Draw 2D pie charts",array("R"=>255,"G"=>255,"B"=>255)); /* Set the default font properties */ $myPicture->setFontProperties(array("FontName"=>"../fonts/Forgotte.ttf","FontSize"=>10,"R"=>80,"G"=>80,"B"=>80)); /* Enable shadow computing */ $myPicture->setShadow(TRUE,array("X"=>2,"Y"=>2,"R"=>0,"G"=>0,"B"=>0,"Alpha"=>50)); /* Create the pPie object */ $PieChart = new pPie($myPicture,$MyData); /* Draw a simple pie chart */ $PieChart->draw2DPie(120,125,array("SecondPass"=>FALSE)); /* Draw an AA pie chart */ $PieChart->draw2DPie(340,125,array("DrawLabels"=>TRUE,"LabelStacked"=>TRUE,"Border"=>TRUE)); /* Draw a splitted pie chart */ $PieChart->draw2DPie(560,125,array("WriteValues"=>PIE_VALUE_PERCENTAGE,"DataGapAngle"=>10,"DataGapRadius"=>6,"Border"=>TRUE,"BorderR"=>255,"BorderG"=>255,"BorderB"=>255)); /* Write the legend */ $myPicture->setFontProperties(array("FontName"=>"../fonts/pf_arma_five.ttf","FontSize"=>6)); $myPicture->setShadow(TRUE,array("X"=>1,"Y"=>1,"R"=>0,"G"=>0,"B"=>0,"Alpha"=>20)); $myPicture->drawText(120,200,"Single AA pass",array("DrawBox"=>TRUE,"BoxRounded"=>TRUE,"R"=>0,"G"=>0,"B"=>0,"Align"=>TEXT_ALIGN_TOPMIDDLE)); $myPicture->drawText(440,200,"Extended AA pass / Splitted",array("DrawBox"=>TRUE,"BoxRounded"=>TRUE,"R"=>0,"G"=>0,"B"=>0,"Align"=>TEXT_ALIGN_TOPMIDDLE)); /* Write the legend box */ $myPicture->setFontProperties(array("FontName"=>"../fonts/Silkscreen.ttf","FontSize"=>6,"R"=>255,"G"=>255,"B"=>255)); $PieChart->drawPieLegend(380,8,array("Style"=>LEGEND_NOBORDER,"Mode"=>LEGEND_HORIZONTAL)); /* Render the picture (choose the best way) */ $myPicture->autoOutput("pictures/example.draw2DPie.png");
phpCHART
With phpChart, you can easily create charts and graphs with powerful features. You have the flexibility to dynamically plot your chart in real-time without user intervention using javascript.
$s1 = array(11, 9, 5, 12, 14); $s2 = array(6, 8, 7, 13, 9); $pc = new C_PhpChartX(array($s1, $s2),'basic_chart'); $pc->set_animate(true); $pc->set_title(array('text'=>'Basic Chart')); $pc->set_series_default(array( 'renderer'=>'plugin::BarRenderer', 'rendererOptions'=>array('sliceMargin'=>2,'innerDiameter'=>110,'startAngle'=>-90,'highlightMouseDown'=>true), 'shadow'=>true )); $pc->add_plugins(array('highlighter', 'canvasTextRenderer')); //set phpChart grid properties $pc->set_grid(array( 'background'=>'lightyellow', 'borderWidth'=>0, 'borderColor'=>'#000000', 'shadow'=>true, 'shadowWidth'=>10, 'shadowOffset'=>3, 'shadowDepth'=>3, 'shadowColor'=>'rgba(230, 230, 230, 0.07)' )); //set axes $pc->set_axes(array( 'xaxis'=>array('rendnerer'=>'plugin::CategoryAxisRenderer'), 'yaxis'=>array('padMax'=>2.0))); //set axes $pc->set_xaxes(array( 'xaxis' => array( 'borderWidth'=>2, 'borderColor'=>'#999999', 'tickOptions'=>array('showGridline'=>false)) )); $pc->set_yaxes(array( 'yaxis' => array( 'borderWidth'=>0, 'borderColor'=>'#ffffff', 'autoscale'=>true, 'min'=>'0', 'max'=>20, 'numberTicks'=>21, 'labelRenderer'=>'plugin::CanvasAxisLabelRenderer', 'label'=>'Energy Use') )); //set legend properties $pc->set_legend(array( 'renderer' => 'plugin::EnhancedLegendRenderer', 'show' => true, 'location' => 'e', 'placement' => 'outside', 'yoffset' => 30, 'rendererOptions' => array('numberRows'=>2), 'labels'=>array('Oil', 'Renewables') )); $pc->draw();
You can effortlessly switch between different types of chart renderers by simply prefixing plugin:: before a renderer name. The most popularly used charts such as line, bar, and pie charts are also well-supported. What’s more, developing animated charts is a breeze – all you need to do is include just one line of code to enable or disable animation in your graph.
Additionally, for debugging purposes, you can activate DEBUG mode which reveals the generated client-side javascript and lists the plugins in use. Finally, the zoom function lets you quickly zoom into graphs using set_cursor(), setting ‘zoom’ property to true.
All these make it effortless to manipulate and create great-looking visual presentations with phpChart!
JpGraph
JpGraph is an impressive open-source graphing library that has the capability to create a wide range of plots, including line and area plots, bar plots, pie charts, scatter plots, field graphs, splines, geo maps, and a variety of 3D effects.
It stands out from other libraries due to its support for anti-aliasing for Pie charts and more advanced formatting of graph titles with 3D Bevel effects. Moreover, JpGraph also offers several more features such as gradient fills for bars being displayed in the legend along with the ability to customize various formatting elements within the legend itself by creating multiple columns.
//-------------------------------------- // Graph 1 //-------------------------------------- $graph1 = new Graph(...); ... [Code to create graph1] ... //-------------------------------------- // Graph 2 //-------------------------------------- $graph2 = new Graph(...); ... [Code to create graph2] ... //-------------------------------------- // Create a combined graph //-------------------------------------- $mgraph = new MGraph(); $xpos1=3;$ypos1=3; $xpos2=3;$ypos2=200; $mgraph->Add($graph1,$xpos1,$ypos1); $mgraph->Add($graph2,$xpos2,$ypos2); $mgraph->Stroke();
Charts 4 PHP
Charts 4 PHP is a powerful, web-based charting tool that makes it easy to create visualizations of your data. By using Charts 4 PHP Framework, crafting interactive database-driven HTML5 dashboards no longer needs to be a lengthy process.
With the Charts 4 PHP API, you can deliver cross-platform and device-compatible charts very quickly – greatly reducing your development time. Drawing on real-time databases including MySQL, MS SQL Server, Oracle, PostgreSQL, and DB2, you can quickly populate phpCHART with your custom SQL query and render the charts in no time.
define("CHARTPHP_DBTYPE","pdo"); define("CHARTPHP_DBHOST","mysql:host=localhost;dbname=testdb"); define("CHARTPHP_DBUSER","username"); define("CHARTPHP_DBPASS","password"); define("CHARTPHP_DBNAME",""); include("../../lib/inc/chartphp_dist.php"); $chart = new chartphp(); $chart->data_sql = "select c.categoryname, sum(a.quantity) as 'Sales 1997', sum(a.quantity)+1000 as 'Sales 1998' from products b, `order details` a, categories c where a.productid = b.productid and c.categoryid = b.categoryid group by c.categoryid order by c.categoryid"; $chart->chart_type = "bar"; $output = $chart->render("c1");
Furthermore, there are a variety of chart types that are all rendered with HTML5 and come with an array of interactive features like animations, tooltips, clickable legends, and more!
What’s more, charts can also be generated on demand directly from CSV files and PHP array data without needing to access a database at all.
Fusion Charts
The Fusion Charts PHP Module makes it easy to create a range of informative and interactive chart types, from Line Charts and Pie Charts to Gantt Charts 150+ more. Unlike other options, this module gives your charts an incredibly responsive quality that can be interacted with on all screen sizes.
include("includes/fusioncharts.php"); $columnChart = new FusionCharts("column2d", "ex1", "100%", 400, "chart-1", "json", '{ "chart": { "caption": "Countries With Most Oil Reserves [2017-18]", "subcaption": "In MMbbl = One Million barrels", "xaxisname": "Country", "yaxisname": "Reserves (MMbbl)", "numbersuffix": "K", "theme": "fusion" }, "data": [ { "label": "Venezuela", "value": "290" }, { "label": "Saudi", "value": "260" }, { "label": "Canada", "value": "180" }, { "label": "Iran", "value": "140" }, { "label": "Russia", "value": "115" }, { "label": "UAE", "value": "100" }, { "label": "US", "value": "30" }, { "label": "China", "value": "30" } ] }'); $columnChart->render();
Forget about manually writing JavaScript methods and objects; you simply construct your app in native PHP or any popular framework like Laravel, CodeIgniter or Symphony then fetch data from your database in order to generate beautiful charts immediately.
This charting library provides features such as dynamically generated JavaScript and HTML code, the ability to load data from MySQL databases into charts, and various methods of adding data such as JSON and XML.
In addition to allowing for drill-downs, animations, and real-time updates, these charts also support zooming and panning so that you can gain powerful insights into the data being displayed.