5 Best PHP Excel Libraries in 2023
Excel is a spreadsheet application that allows users to input and manipulate data. It is part of the Microsoft Office suite of programs and is available for both Windows and Mac users. Excel files are saved with a .xlsx extension and can contain code, formulas, charts, and other spreadsheet elements. Excel is a powerful tool that can be used for a variety of purposes, from tracking household expenses to creating complex financial models.
If you’re looking for a library to help you work with Excel files in PHP, then you’re in luck. There are a number of great options to choose from, and we’ve compiled a list of the 5 best ones for you.
5 Best PHP Excel Libraries
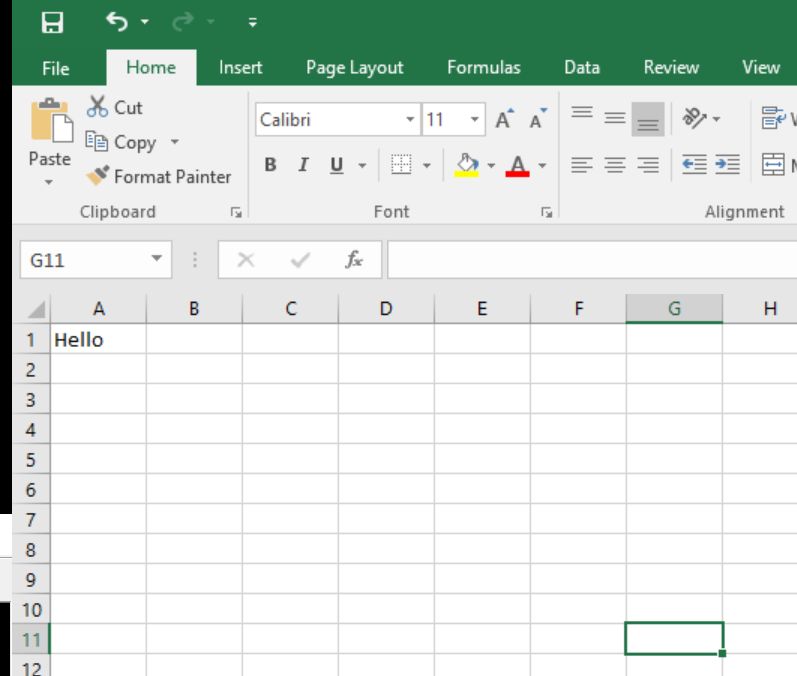
phpspreadsheet
PhpSpreadsheet is a library that offers a powerful set of classes for reading and writing various spreadsheet file formats. No matter the spreadsheet application, PhpSpreadsheet has the power to read and write these files in a standalone way.
PHPSpreadsheet is the newest version of PHPExcel. It is not compatible with the old version, but it is much better because it follows stricter rules for code (namespaces, PSR compliance, use of latest PHP features, etc.).
Format | Reading | Writing |
---|---|---|
Open Document Format/OASIS (.ods) | ✓ | ✓ |
Office Open XML (.xlsx) Excel 2007 and above | ✓ | ✓ |
BIFF 8 (.xls) Excel 97 and above | ✓ | ✓ |
BIFF 5 (.xls) Excel 95 | ✓ | |
SpreadsheetML (.xml) Excel 2003 | ✓ | |
Gnumeric | ✓ | |
HTML | ✓ | ✓ |
SYLK | ✓ | |
CSV | ✓ | ✓ |
PDF (using either the TCPDF, Dompdf or mPDF libraries, which need to be installed separately) | ✓ |
require 'vendor/autoload.php'; use PhpOffice\PhpSpreadsheet\Spreadsheet; use PhpOffice\PhpSpreadsheet\Writer\Xlsx; $spreadsheet = new Spreadsheet(); $sheet = $spreadsheet->getActiveSheet(); $sheet->setCellValue('A1', 'Good morning'); $writer = new Xlsx($spreadsheet); $writer->save('my first excel file.xlsx');
Spout
Whether you need to read or write spreadsheet files in a PHP application, Spout is the library for you. It is capable of processing very large files while keeping memory usage low. So whether your file is 500KB or 500MB, Spout can handle it without breaking a sweat.
Your PHP version must be 7.2 or higher to install Spout. Additionally, the php_zip and php_xmlreader extensions must be enabled on your server.
use Box\Spout\Reader\Common\Creator\ReaderEntityFactory; $reader = ReaderEntityFactory::createReaderFromFile('/path/to/file.ext'); $reader->open($filePath); foreach ($reader->getSheetIterator() as $sheet) { foreach ($sheet->getRowIterator() as $row) { // do stuff with the row $cells = $row->getCells(); ... } } $reader->close();
PHP Excel Templator
This is a PHP spreadsheet library that lets you export Excel documents from an Excel template. You don’t need to write code, create styles, and so on from scratch when utilizing the extension.
The extension allows you to insert variables into your templates, so you can quickly and easily create formatted tables with data. You can also change the cell styles on the fly, making it easy to get the exact look you want.
use alhimik1986\PhpExcelTemplator\PhpExcelTemplator; require_once('vendor/autoload.php'); // if you don't use framework PhpExcelTemplator::saveToFile('./template.xlsx', './exported_file.xlsx', [ '{current_date}' => date('d-m-Y'), '{department}' => 'Sales department', ]);
It supports both one-dimension and 2-dimension arrays, so you can quickly and easily create tables with data. You can also apply the same template to multiple sheets, making it easy to create an entire excel file using a single template.
SimpleXLSX
If you need to work with Microsoft Excel 2007 files in your PHP application, then you’ll want to check out the SimpleXLSX library. It makes it easy to read and write data from Excel XLSx files, without requiring any additional extensions.
use Shuchkin\SimpleXLSX; if ( $xlsx = SimpleXLSX::parse('book.xlsx') ) { print_r( $xlsx->rows() ); } else { echo SimpleXLSX::parseError(); }
This makes it a great choice for applications that need to interoperate with Microsoft Office products. The library is also very lightweight, making it a good choice for applications with limited resources.
PHP_XLSXWriter
PHP_XLSXWriter is designed to be lightweight and has minimal memory usage, making it ideal for larger projects. Additionally, it supports currency/date/numeric cell formatting as well as basic cell styling.
The library supports basic features like PHP 5.2.1+ and UTF-8 encoding. It also allows you to format your cells in different ways, including currency and date formats, as well as numeric formatting.
$header = array( 'created'=>'date', 'product_id'=>'integer', 'quantity'=>'#,##0', 'amount'=>'price', 'description'=>'string', 'tax'=>'[$$-1009]#,##0.00;[RED]-[$$-1009]#,##0.00', ); $data = array( array('2015-01-01',873,1,'44.00','misc','=D2*0.05'), array('2015-01-12',324,2,'88.00','none','=D3*0.05'), ); $writer = new XLSXWriter(); $writer->writeSheetHeader('Sheet1', $header ); foreach($data as $row) $writer->writeSheetRow('Sheet1', $row ); $writer->writeToFile('file.xlsx');
You can also style your cells however you want. It can handle spreadsheets with over 100,000 rows without any problems.