Top 5 Templating Engines for JavaScript
JavaScript is one of the most popular programming languages in the world, and templating engines are a vital part of its development. A templating engine allows you to create reusable chunks of code which can be inserted into HTML documents. This saves time and makes your code more efficient.
There are many different templating engines available for JavaScript, but which one should you choose? In this article, we’ll take a look at the top 5 templating engines for JavaScript and see which one is right for you.
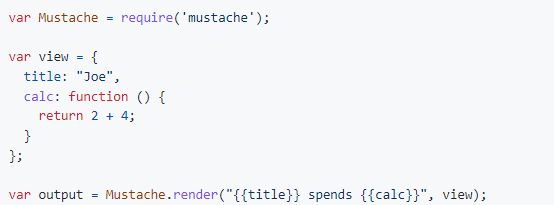
The Best JavaScript Templating Engines
Mustache.js
Mustache.js is a popular templating engine that is used by many major websites, including Twitter and LinkedIn. Mustache.js is easy to learn and use, and it provides a great deal of flexibility when it comes to creating templates. Its logic-less design means that it can be used for any kind of development, without the need for hours of converting data to UI elements work. Instead, Mustache simply convert provided values in a hash or object to tags.
You can use mustache.js in web browsers, server-side environments such as Node.js, and even in CouchDB views. mustache.js ships with support for the CommonJS module API, the Asynchronous Module Definition API (AMD) and ECMAScript modules.
This makes it easy to integrate mustache.js into your existing development workflow. And because mustache.js is open source, you can always be sure that you have the latest and greatest features at your disposal.
var Mustache = require('mustache'); var view = { title: "Joe", calc: function () { return 2 + 4; } }; var output = Mustache.render("{{title}} spends {{calc}}", view);
Handlebars.js
Handlebars.js is another popular templating engine which is used by many websites, including eBay and Tumblr. Handlebars.js is similar to Mustache.js in terms of syntax and usage, but it also provides additional features such as helpers and partials.
var source = "<p>Hello, my name is {{name}}. I am from {{hometown}}. I have " + "{{kids.length}} kids:</p>" + "<ul>{{#kids}}<li>{{name}} is {{age}}</li>{{/kids}}</ul>"; var template = Handlebars.compile(source); var data = { "name": "Alan", "hometown": "Somewhere, TX", "kids": [{"name": "Jimmy", "age": "12"}, {"name": "Sally", "age": "4"}]}; var result = template(data); // Would render: // <p>Hello, my name is Alan. I am from Somewhere, TX. I have 2 kids:</p> // <ul> // <li>Jimmy is 12</li> // <li>Sally is 4</li> // </ul>
It is compatible with Mustache templates, making it easy to swap out one engine for the other. With Handlebars, you can quickly and easily create templates that are both effective and easy to use. Additionally, Handlebars provides a number of helpful features that make templating easier and more streamlined.
EJS
EJS is a templating engine that is designed for use with Node.js applications. EJS is simple to use and provides a number of useful features, such as layout support and locals support.
let template = ejs.compile(str, options); template(data); // => Rendered HTML string ejs.render(str, data, options); // => Rendered HTML string ejs.renderFile(filename, data, options, function(err, str){ // str => Rendered HTML string });
EJS is a templating language that makes it easy to generate HTML markup with JavaScript. It features a simple syntax with a variety of ways to embed JavaScript code in your templates.
With EJS, you can control the flow of your template with <% %> tags, escape variables for safe output with <%= %> tags, and generate raw HTML with <%- %> tags. You can also enable newline-trim mode (‘newline slurping’) by ending your tag with -%>, or whitespace-trim mode (which slurps all whitespace) by using <%_ _%> instead of <% %>.
In addition, EJS supports custom delimiters so you can use any characters you like to delineate your code. Finally, EJS is fully compatible with the Express view system, and offers static caching of intermediate JavaScript and templates for performance.
Jade
Jade is a high performance template engine that is heavily influenced by Haml. It is designed for use with Node.js applications.
doctype html html(lang="en") head title= pageTitle script(type='text/javascript'). if (foo) bar(1 + 5) body h1 Jade - node template engine #container.col if youAreUsingJade p You are amazing else p Get on it! p. Jade is a terse and simple templating language with a strong focus on performance and powerful features.
It is implemented with JavaScript for Node and is a clean, whitespace sensitive syntax for writing HTML. Jade provides an easy way to create templates that are both well-formed and easy to read. Additionally, Jade offers a number of features that make it an attractive option for template development. For example, Jade supports inline JavaScript, which allows you to embed JavaScript code directly into your templates.
This makes it easy to create dynamic content that can be updated on the fly. Additionally, Jade supports partials, which allow you to reuse common template fragments in multiple places. This can be a huge time saver when you need to create similar pages or sections of a site.
Nunjucks
Nunjucks is a templating engine that was created by Mozilla. Nunjucks is similar to Jade in terms of syntax and usage, but it also provides additional features such as autoescaping and extensibility.
{% extends "base.html" %} {% block header %} <h1>{{ title }}</h1> {% endblock %} {% block content %} <ul> {% for name, item in items %} <li>{{ name }}: {{ item }}</li> {% endfor %} </ul> {% endblock %}
It features a powerful language with block inheritance, autoescaping, macros, asynchronous control, and more. Plus, it’s heavily inspired by jinja2 so you’ll be right at home if you’re already familiar with that templating language. And best of all, it’s fast and lean.
The runtime is only 8K gzipped and it comes with precompiled templates so you can use it in the browser without any issues. Plus, it’s highly extensible so you can add your own custom filters and extensions. And finally, it’s available everywhere. You can use it in node or in any modern web browser.