How to Detect OS Using JavaScript
It’s not a secret that most of the devices in our world are running on different operating systems. Some are MacOS, some are iOS, Windows, and so on. There is also Linux OS which can be detected as well. Detecting the operating system with JavaScript is actually quite easy to do.
The easiest way to detect the operating system is by using the window.navigator object. This object contains a lot of information about the browser and the user agent. We can use this information to detect the operating system.
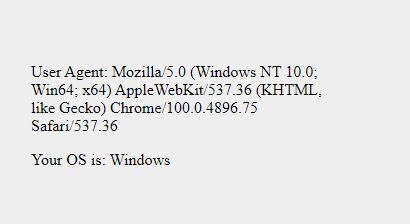
<div id="useragent"></div> <p></p> <div id="result"></div>
var userAgent = window.navigator.userAgent, platform = window.navigator?.userAgentData?.platform ?? window.navigator.platform, macosPlatforms = ['Macintosh', 'MacIntel', 'MacPPC', 'Mac68K'], windowsPlatforms = ['Win32', 'Win64', 'Windows', 'WinCE'], iosPlatforms = ['iPhone', 'iPad', 'iPod']; var platformDisplay = "Your OS is: "; if (iosPlatforms.indexOf(platform) !== -1) { platformDisplay += 'iOS'; } else if (/Android/.test(userAgent)) { platformDisplay += 'Android'; } else if (windowsPlatforms.indexOf(platform) !== -1) { platformDisplay += 'Windows'; } else if (macosPlatforms.indexOf(platform) !== -1) { platformDisplay += 'macOS'; } else if (!platform && /Linux/.test(platform)) { platformDisplay += 'Linux'; } document.querySelector("#useragent").innerHTML = "<div>User Agent: " + userAgent + "</div>"; document.querySelector("#result").innerText = platformDisplay;