How to Create Dynamic HTML Pages with JavaScript?
A dynamic web page changes its content in response to user input or some other event. For example, a news website might display the latest headlines on its home page, and then change those headlines when new stories are added. A shopping website might show a list of products on its home page, and then change the products as new items are added to the inventory.
With JavaScript, you can change the content of an HTML page without having to reload the page in the browser. This makes for a more responsive and interactive user experience.
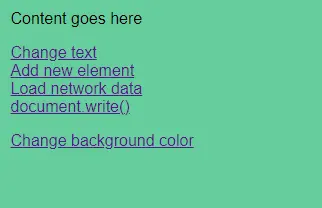
We have several approaches to creating a dynamic page such as adding DOM elements, changing text on a user’s event, and loading network data.
<div id="wrapper"> <div id="content">Content goes here</div> <div class="btn-group mt"> <div><a class="btn" href="#" onClick="changeText()">Change text</a></div> <div><a class="btn" href="#" onClick="addNewElements()">Add new element</a></div> <div><a class="btn" href="#" onClick="loadNetworkData()">Load network data</a></div> <div><a class="btn" href="#" onClick="writeDocument()">document.write()</a></div> </div> <div class="btn-group mt"> <div><a class="btn" href="#" onClick="changeBackgroundColor()">Change background color</a></div> </div> </div> <script type="text/javascript"> function changeText(){ document.querySelector('#content').textContent = 'New text added by button.'; } function addNewElements(){ const ul = document.createElement('ul'); const li1 = document.createElement('li'); li1.innerText = 'Item 1'; ul.appendChild(li1); const li2 = document.createElement('li'); li2.innerText = 'Item 2'; ul.appendChild(li2); document.querySelector('#content').appendChild(ul); } function loadNetworkData(){ fetch('https://jsonplaceholder.typicode.com/posts') .then(response => response.json()) .then(function(result) { //var objs = json.map(JSON.parse); const ul = document.createElement('ul'); for(var i = 0; i <= 10 ; i++){ let li = document.createElement('li'); li.innerText = result[i].title; ul.appendChild(li); } document.querySelector('#content').appendChild(ul); }); } function writeDocument(){ document.open(); document.write('<h1>writeDocument() method</h1>'); document.close(); } function changeBackgroundColor(){ let randomColor = "#"+(Math.random()*16777215|0).toString(16); document.querySelector('#wrapper').style.background = randomColor; }