JavaScript Boilerplates: Plugin Structure, Best Frameworks
If you’re a web developer, then you know that boilerplate code is a lifesaver. It can help you get up and running with a project quickly, without having to spend time writing all the necessary code from scratch. And when it comes to JavaScript, there are plenty of boilerplate codes to choose from.
JavaScript Boilerplates
JavaScript Plugin Boilerplate
In software development, a boilerplate is a unit of code that can be reused over and over again. A JavaScript plugin boilerplate is a boilerplate specifically for creating JavaScript plugins. Plugin boilerplates typically include code for setting up the plugin, including the creation of variables and functions, as well as code for customizing the plugin to fit your needs.
In addition, they often include comments and documentation to help you understand how the plugin works. By using a plugin boilerplate, you can save yourself a lot of time and effort in creating your own plugins. In addition, you can be confident that your plugins will be well-organized and easy to maintain.
Simple version:
var myPlugin = (function () { 'use strict'; var publicMethods = {}; var defaults = { loop: true, counterStart: 1, }; var aPrivateMethod = function () { }; publicMethods.doSomething = function () { //call private method or do other things here aPrivateMethod(); }; publicMethods.init = function (options) { var settings = extend( defaults, options || {} ); }; return publicMethods; })();
More complicated version: view here.
Best JavaScript Frameworks
React
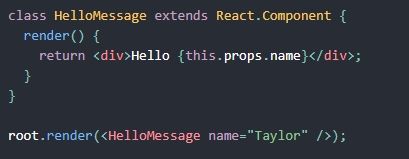
React is a declarative, efficient, and fast JavaScript library for building user interfaces. It is Declarative, Virtual DOM, Event handling, and JSX. React makes it easy to create interactive and dynamic UIs for websites and mobile applications. The Declarative views make the code readable and easy to debug.
With React, you can create reusable components so that your code is easy to read and maintain. Because React uses a virtual DOM, it updates the view very quickly when data changes. This makes your website or app feel more responsive to users. React also has a cross-browser event system, so your code will work consistently across different browsers.
JSX is a markup syntax that closely resembles HTML. JSX makes writing React components easier by making the syntax almost HTML-like. You can use React without using JSX but using JSX makes React more fun and easy to use.
React Native is a custom renderer for React that uses native components instead of web components like React. This makes your app more responsive and faster because it doesn’t have to re-render the entire page every time there’s a chance. React Native also has a cross-browser event system, so your code will work consistently across different browsers.
Vue.js
Vue.js is a JavaScript framework for developing user interfaces and single-page applications. It provides declarative templates and powerful reactive data binding which makes it an ideal choice for complex, dynamic web applications. Vue.js is smaller, faster, and easier to learn than other frameworks such as Angular or React. It is also more modular, making it easy to combine with other libraries or frameworks if needed.
It was created by Evan You, who worked on Google Maps and Gmail Verification before joining Google Creative Lab. Vue.js features an incrementally adaptable architecture that focuses on declarative rendering and component composition. Advanced features include scoped CSS, powerful directives, transition effects, animations, server-side rendering, arrives tooling, and supporting libraries.
Vue is mainly used for building user interfaces and single-page applications. The core feature of Vue is the Virtual DOM that syncs with the real DOM. Vue also provides a two-way binding between the HTML template and the JavaScript code. When a data property changes, it updates the corresponding parts of the DOM tree automatically.
The advantage of using Vue over other frameworks is its simplicity. The syntax is easy to understand, and a developer can build small to large applications without any issues. Moreover, the performance of Vue is really good because it updates only those parts of the DOM that have changed.
This makes it very fast when compared to other frameworks that update the entire DOM tree on every change. Another good thing about Vue is that it comes with various tools and libraries that make development easier.
Backbone.js
Backbone.js is a javascript library that gives structure to web applications by providing models with key-value binding and custom events, collections with a rich API of enumerable functions, views with declarative event handling, and connects it all to your existing API over a RESTful JSON interface. Backbone has a free and open-source library and contains over 100 available extensions. Backbone has a soft dependency on jQuery and a hard dependency on Underscore.js.
Backbone lets you create client-side web applications or mobile applications in a well-structured way. With Backbone, you can focus on application logic without having to worry about the low-level details of managing your data or views. Backbone’s collection of libraries and extensions makes it easy to get started with Backbone and start building sophisticated web applications quickly.
Node.js
Boilerplate code for Node.js is a great starting point for web applications or APIs. It includes everything you need to get started, including a simple server, routing, and database access. Node.js was developed by Ryan Dahl in 2009, and its features include being fast, asynchronous, and event-driven I/O, single-threaded, and highly scalable.
Node.js is built on the V8 JavaScript engine of Google Chrome, which makes code execution fast. The event mechanism of Node.js enables the server to respond in a non-blocking manner, which makes it highly scalable. When it comes to programming, Node.js has an advantage over traditional servers because it uses an event-driven model which makes it easier to program complex applications.
Another reason why Node.js is popular is that it runs on multiple platforms including Windows, Linux, and Mac OS X.Node.js also has a large community of developers who contribute to the development of the platform and create modules that can be used by others.
Mithril
Mithril.js is a client-side JavaScript framework for building Single Page Applications. Mithril.js is a cutting-edge client-side JavaScript framework that is rapidly gaining popularity among developers.
One of the benefits of Mithril is its small code size; at just 7.8 kB gzipped, it’s one of the smallest frameworks available. In addition, Mithril has no dependencies on other libraries, which makes it easy to include in your project. Another benefit of Mithril is its declarative syntax.
It offers a small, fast package that provides routing and XHR utilities out of the box. This makes it ideal for building Single Page Applications. In addition, Mithril.js has excellent browser support, covering IE11, Firefox ESR, and the last two versions of Firefox, Edge, Safari, and Chrome.
Many large companies are already using Mithril.js, such as Vimeo and Nike. It is also being used by open-source platforms like Lichess.
Meteor
Meteor is a full-stack JavaScript platform for developing web and mobile applications. It includes everything you need to get started, including templates, libraries, and a debugger.
Meteor makes it easy to create maintainable code with its reactive programming model, which allows you to update your application’s state in real-time as data changes. This ensures that all users see the latest changes as soon as they are made. Meteor also integrates well with other technologies, making it easy to connect to backend databases or use front-end frameworks like React or AngularJS.
It integrates with technologies you already use and provides popular frameworks and tools out-of-the-box so you can focus on building features instead of configuring disparate components yourself. Meteor also allows you to build apps for any device with the same codebase for a seamless update experience for your users. Over 500k developers trust Meteor because it is a mature framework that has been developed for over a decade by industry giants.
Ember.js
Ember.js is a powerful, productive, battle-tested JavaScript framework that makes it easy to build modern web applications. With Ember.js, you get everything you need to create rich UIs that work on any device. Ember CLI provides code generation and scaffolding so you can focus on building your app, not configuring it.
The router seamlessly supports nested URLs with incremental data fetching, making sure your users always have the latest information. And while you can use any data layer for your app, every new Ember app includes the fully-featured Ember Data library.
AngularJS
AngularJS is a structural framework for dynamic web apps. It lets you use HTML as your template language and lets you extend HTML’s syntax to express your application’s components clearly and succinctly. AngularJS’s data binding and dependency injection eliminate much of the code you would otherwise have to write. And it all happens within the browser, making it an ideal partner with any server technology.
AngularJS is what HTML would have been, had it been designed for applications. HTML is a great declarative language for static documents. It does not contain much in the way of creating applications, and as a result building web applications is an exercise in what do I have to do to trick the browser into doing what I want?
AngularJS lets you extend HTML vocabulary for your application. The resulting environment is extraordinarily expressive, readable, and quick to develop.