How to Update TextField on Button Click in Flutter
When it comes to updating the text inside a text field in Flutter, there is a TextEditingController that supports this. If you want to update the text inside a text field in Flutter, then you can use the controller property of the TextField widget.
All you need to do is call TextField’s controller property and set it equal to the new value that you want to display.
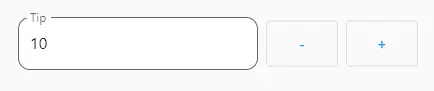
The example below is a widget with plus and minus buttons that add and subtract the value of the number field.
class TipField extends StatefulWidget { const TipField({Key? key}) : super(key: key); @override State<TipField> createState() => _TipFieldState(); } class _TipFieldState extends State<TipField> { final TextEditingController _controller = TextEditingController(); @override void dispose() { _controller.dispose(); super.dispose(); } @override Widget build(BuildContext context) { return Row( children: [ Flexible( flex: 6, child: TextField( controller: _controller, onChanged: (value) { }, keyboardType: const TextInputType.numberWithOptions(decimal: true, signed: false), decoration: InputDecoration( label: const Text("Tip"), border: OutlineInputBorder( borderRadius: BorderRadius.circular(12.0), ), filled: true, fillColor: Colors.white, ), ), ), Flexible( flex: 2, child: Padding( padding: const EdgeInsets.only(left: 8.0), child: OutlinedButton( onPressed: () { double amount = double.tryParse(_controller.text) ?? 0; amount = amount == 0 ? 0 : amount - 1; _controller.text = amount.toString(); }, child: const SizedBox(height: 46, child: Center(child: Text("-"))), ), ), ), Flexible( flex: 2, child: Padding( padding: const EdgeInsets.only(left: 8.0), child: OutlinedButton( onPressed: () { double amount = double.tryParse(_controller.text) ?? 0; _controller.text = (amount + 1).toString(); }, child: const SizedBox(height: 46, child: Center(child: Text("+"))), ), ), ), ], ); } }