How to Set Gradient Background Color for a Screen in Flutter
There are many different ways to customize the appearance of your Flutter app, and one method that is particularly effective is setting a gradient background color for your entire screen. This helps to create a dynamic and visually interesting experience for users, while also highlighting important elements like buttons or content blocks within the interface.
To set a gradient background color in Flutter, simply wrap your Scaffold with a Container widget then choose a base color using the provided palette tool, or by inputting RGB values manually for its decoration.
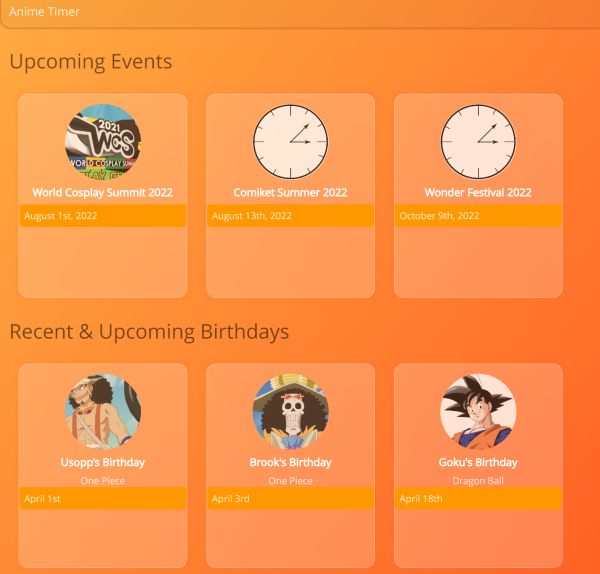
import 'package:anime_timer/constant/theme.dart'; import 'package:anime_timer/ui/common/appbar.dart'; import 'package:flutter/material.dart'; class MyScaffold extends StatelessWidget { final Widget? child; final List<Widget>? action; final Widget? floatingActionButton; const MyScaffold({Key? key, this.child, this.action, this.floatingActionButton}) : super(key: key); @override Widget build(BuildContext context) { return Container( decoration: const BoxDecoration( gradient: LinearGradient( begin: Alignment.topLeft, end: Alignment.bottomRight, colors: [Colors.orangeAccent, Colors.deepOrange], )), child: Scaffold( appBar: MainAppBar(action: action ?? []), body: SafeArea( child: SingleChildScrollView( child: Padding( padding: const EdgeInsets.all(16.0), child: SizedBox( width: MediaQuery.of(context).size.width, child: child!, ), ), ), ), floatingActionButton: floatingActionButton, ), ); } }