How to Create Rounded Input TextField without Border in Flutter?
A TextField in Flutter is a basic input field that allows users to enter text. In this recipe, we’ll create a simple rounded TextField that doesn’t have a border around it.
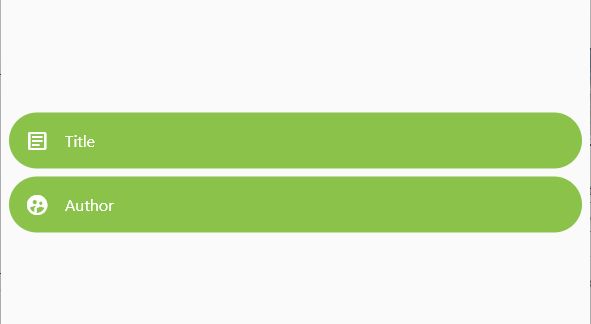
RoundedTextField( controller: _controller, hintText: "Title", icon: Icons.article_outlined, backgroundColor: Colors.lightGreen, onChanged: (value) { print(value); }, ), RoundedTextField( controller: _controller, hintText: "Author", icon: Icons.supervised_user_circle, color: Colors.white, backgroundColor: Colors.lightGreen, onChanged: (value) { print(value); }, ),
class RoundedTextField extends StatelessWidget { final String? hintText; final IconData? icon; final Color? color; final Color? backgroundColor; final ValueChanged<String>? onChanged; final TextEditingController? controller; const RoundedTextField({Key? key, this.hintText, this.icon, this.onChanged, this.color = Colors.white, this.backgroundColor = Colors.blueAccent, this.controller}) : super(key: key); @override Widget build(BuildContext context) { return Container( padding: const EdgeInsets.symmetric(horizontal: 16, vertical: 4), decoration: BoxDecoration( color: backgroundColor, borderRadius: BorderRadius.circular(36), ), child: TextField( controller: controller, onChanged: onChanged, cursorColor: color, decoration: InputDecoration( icon: Icon( icon, color: color, ), hintText: hintText, hintStyle: TextStyle(color: color), border: InputBorder.none, ), ), ); } }