How to Create Responsive Layout in Flutter?
Responsive layout is very important in mobile app development and website design. It ensures that your content looks good and is easy to read on any device, whether it’s a phone, tablet, or desktop computer. Without a responsive layout, your app or website could look cluttered and unprofessional.
Fortunately, creating a responsive layout with Flutter is easy thanks to the LayoutBuilder. The LayoutBuilder class builds a widget tree that can depend on the parent widget’s size. This is useful when you want your children’s final layouts to match their intrinsic sizes, but don’t need access to all of those calculations in order to make this happen!
If you want to create a truly responsive app or website, it’s important to test your design on different devices. This will help you ensure that your content looks good and is easy to use no matter what device your users are using.
Here is the widget we will use to set mobile or desktop layout depending on the breakpoint:
import 'package:flutter/material.dart'; class ResponsiveLayout extends StatelessWidget { final Widget? mobileLayout; final Widget? desktopLayout; final double breakPoint = 640.0; const ResponsiveLayout({Key? key, this.mobileLayout, required this.desktopLayout}) : super(key: key); @override Widget build(BuildContext context) { return LayoutBuilder( builder: (context, constraints) { if (constraints.maxWidth >= breakPoint) { return desktopLayout!; } else { return mobileLayout ?? desktopLayout!; } }, ); } }
Example:
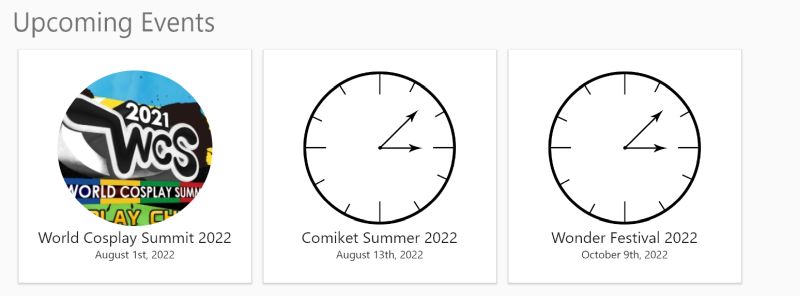
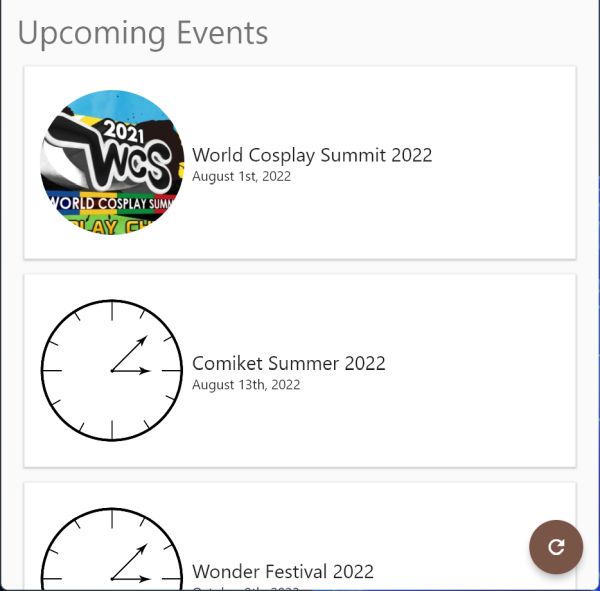
ResponsiveLayout( desktopLayout: Wrap( runSpacing: 16, children: [ ...events.map((e) => EventCard(event: e)) ], ), mobileLayout: Column( mainAxisAlignment: MainAxisAlignment.start, crossAxisAlignment: CrossAxisAlignment.start, children: [ ...events.map((e) => MobileEventCard(event: e)) ], ), )