How to Create an Autocomplete Field in Flutter
An autocomplete field is a great way to help users input data quickly and accurately. By providing a list of suggested values, an autocomplete field can help reduce typos and speed up data entry. Autocomplete fields are especially useful when entering data into forms, as they can help ensure that all required fields are filled out correctly. Creating an autocomplete field in Flutter is relatively simple and can be accomplished with the built-in Autocomplete widget.
In Flutter, the Autocomplete widget can help you make a selection from a list of options by entering some text into a field. The text input is received in a field built with the fieldViewBuilder parameter, and the options to be displayed are determined using the optionsBuilder. The options are then rendered with the optionsViewBuilder.
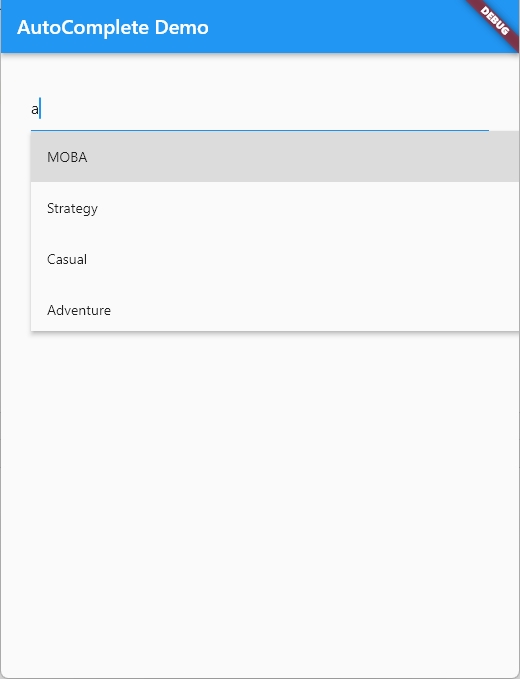
import 'package:flutter/material.dart'; class ExamplePage extends StatefulWidget { const ExamplePage({Key? key}) : super(key: key); @override State<ExamplePage> createState() => _ExamplePageState(); } class _ExamplePageState extends State<ExamplePage> { final List<String> _options = <String>[ 'RPG', 'MOBA', 'Strategy', 'Casual', 'Adventure', 'Simulation', 'City Building', 'Tower Defense', ]; @override Widget build(BuildContext context) { return Scaffold( appBar: AppBar( title: const Text('AutoComplete Demo'), ), body: Container( padding: const EdgeInsets.all(30), child: Autocomplete<String>( optionsBuilder: (TextEditingValue textEditingValue) { if (textEditingValue.text == '') { return List<String>.empty(); } return _options.where((String option) { return option.toLowerCase().contains(textEditingValue.text.toLowerCase()); }); }, onSelected: (String selection) { debugPrint('Selected: $selection'); }, ), ), ); } }