How to Create a Notched Bottom Bar in Flutter?
Notched Bottom Bars are a type of Bottom App Bar that has an opening in the middle to accommodate a Floating Action Button (FAB). It is often used in conjunction with a Scaffold.
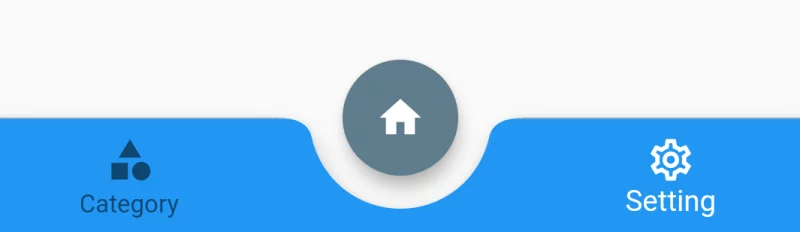
import 'package:flutter/material.dart'; class HomePage extends StatefulWidget { @override _HomePageState createState() => _HomePageState(); } class _HomePageState extends State<HomePage> { int _currentIndex = 1; List<Widget> _pages = List<Widget>(); @override void initState() { _pages.add(CategoryScreen()); _pages.add(HomeScreen()); _pages.add(SettingScreen()); super.initState(); } @override Widget build(BuildContext context) { return Scaffold( appBar: AppBar(title: Text('BottomNavigationBar')), body: _pages[_currentIndex], bottomNavigationBar: BottomAppBar( shape: CircularNotchedRectangle(), notchMargin: 8.0, clipBehavior: Clip.antiAlias, child: Container( height: kBottomNavigationBarHeight, child: Container( decoration: BoxDecoration( color: Colors.white, border: Border( top: BorderSide( color: Colors.grey, width: 0.5, ), ), ), child: BottomNavigationBar( currentIndex: _currentIndex, backgroundColor: Colors.blue, selectedItemColor: Colors.white, onTap: (index) { setState(() { _currentIndex = index; }); }, items: [ BottomNavigationBarItem( icon: Icon(Icons.category), label: 'Category'), BottomNavigationBarItem(icon: Icon(Icons.home), label: ''), BottomNavigationBarItem( icon: Icon(Icons.settings_outlined), label: 'Setting') ]), ), ), ), floatingActionButtonLocation: FloatingActionButtonLocation.miniCenterDocked, floatingActionButton: Padding( padding: const EdgeInsets.all(8.0), child: FloatingActionButton( backgroundColor: _currentIndex == 1 ? Colors.blue : Colors.blueGrey, child: Icon(Icons.home), onPressed: () => setState(() { _currentIndex = 1; }), ), ), ); } } class HomeScreen extends StatelessWidget { @override Widget build(BuildContext context) { return Container( width: double.infinity, height: double.infinity, child: Center(child: Text('Home')), ); } } class CategoryScreen extends StatelessWidget { @override Widget build(BuildContext context) { return Container( width: double.infinity, height: double.infinity, child: Center(child: Text('Category')), ); } } class SettingScreen extends StatelessWidget { @override Widget build(BuildContext context) { return Container( width: double.infinity, height: double.infinity, child: Center(child: Text('Settings')), ); } }