How to Create a Countdown in Flutter
A countdown timer can be a great way to add urgency or excitement to your app. For example, you might use a countdown timer to create a sense of urgency for a sale or promotion or to build excitement for an upcoming event.
There are many ways to use a countdown timer in your app. Here are a few ideas:
- Include a countdown timer in a marketing app, showing the time remaining until a sale or promotion ends.
- Display a countdown timer on your website to show when an event is starting, such as a new product launch or webinar.
- Use a countdown timer in your app to build anticipation for upcoming updates or features.
In this snippet, we will use flutter_countdown_timer package to implement countdown. It is easy to customize the UI for the countdown.
flutter_countdown_timer: ^4.1.0
//simple usage CountdownTimer( endTime: DateTime.now().millisecondsSinceEpoch + 1000 * 60, ),
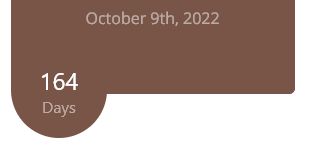
class CountDownTimeWidget extends StatefulWidget { final int? endTime; const CountDownTimeWidget({Key? key, required this.endTime}) : super(key: key); @override State<CountDownTimeWidget> createState() => _CountDownTimeWidgetState(); } class _CountDownTimeWidgetState extends State<CountDownTimeWidget> { @override Widget build(BuildContext context) { return CountdownTimer( endTime: widget.endTime!, widgetBuilder: (_, CurrentRemainingTime? time) { if (time == null) { return Container(); } return Text("${time.hours.toString().padLeft(2, "0")}:${time.min.toString().padLeft(2, "0")}:${time.sec.toString().padLeft(2, "0")}", style: const TextStyle( fontSize: 18, )); }, ); } }
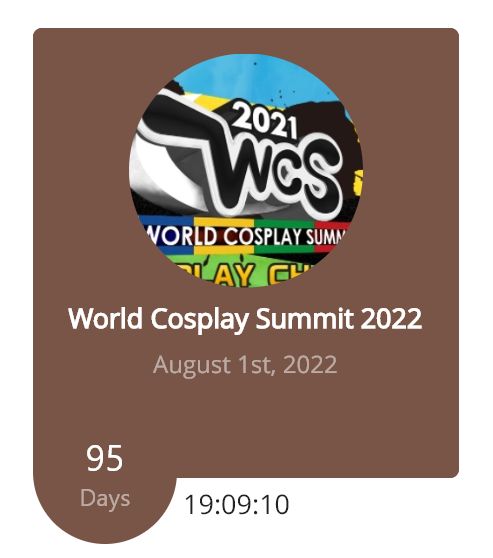
class CountDownDaysWidget extends StatefulWidget { final int? endTime; const CountDownDaysWidget({Key? key, required this.endTime}) : super(key: key); @override State<CountDownDaysWidget> createState() => _CountDownDaysWidgetState(); } class _CountDownDaysWidgetState extends State<CountDownDaysWidget> { @override Widget build(BuildContext context) { return CountdownTimer( endTime: widget.endTime!, widgetBuilder: (_, CurrentRemainingTime? time) { if (time == null) { return Container(); } return Column( children: [ CircleAvatar( child: Column( mainAxisAlignment: MainAxisAlignment.center, crossAxisAlignment: CrossAxisAlignment.center, children: [ Text( "${time.days}", style: TextStyle(fontSize: 24, color: MyTheme.cardTextColor), ), Text( 'Days', style: TextStyle(color: MyTheme.cardTextColor.withOpacity(0.5)), ), ], ), radius: MyTheme.countdownDayCircleRadius, backgroundColor: MyTheme.cardColor, ), ], ); }, ); } }