How to Change Background Color of a Screen/Scaffold in Flutter
If you are looking to change the background color on a screen in Flutter, there are a few different ways that you can do this. In this blog post, we will show you how to use two different methods to change the background color of a screen.
Scaffold backgroundColor propety
The Scaffold is a very useful and widely used Widget in Flutter Apps. Scaffold also provides a property called backgroundColor to change its background color. The following code snippet shows how you can use this property to change the background color of your screen.
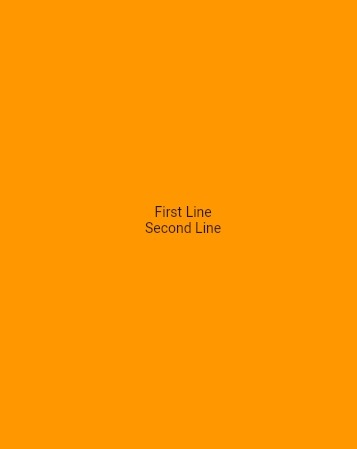
In the following code, we use backgroundColor: Colors.orange to set oraneg as the screen’s background color.
class MyApp extends StatelessWidget { @override Widget build(BuildContext context) { return MaterialApp( debugShowCheckedModeBanner: false, home: Scaffold( backgroundColor: Colors.orange, body: Center( child: Column( mainAxisAlignment: MainAxisAlignment.center, crossAxisAlignment: CrossAxisAlignment.center, children: [ const Text("First Line"), const Text("Second Line"), ], ), ), ), ); } }
ThemeData class
ThemeData is a class that defines the configuration of how your app looks. The theme can be used to configure all aspects, such as colors and fonts in one place instead of having them spread across many different files with varying styles; making updates much easier when you want to change something around without needing an entire app update.
Some widgets should look like they fit in with the overall theme. You can use the current theme’s configuration to make them look that way. The colorScheme and textTheme properties will always have values, so you can rely on them.
ThemeData’s scaffoldBackgroundColor property determines the whole app’s scaffold’s background color. This approach is better if you want to modify all screens.
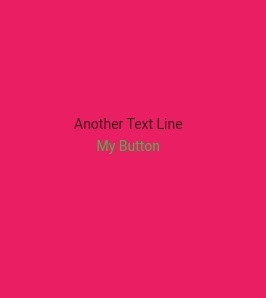
class MyApp extends StatelessWidget { @override Widget build(BuildContext context) { return MaterialApp( debugShowCheckedModeBanner: false, theme: ThemeData( primarySwatch: Colors.green, scaffoldBackgroundColor: Colors.pink, ), home: Scaffold( body: Center( child: Column( mainAxisAlignment: MainAxisAlignment.center, crossAxisAlignment: CrossAxisAlignment.center, children: [ const Text("Another Text Line"), TextButton(onPressed: (){ }, child: Text("My Button")) ], ), ), ), ); } }