How to Add Wave Animation in Flutter
Wave animation is a popular effect used in mobile apps and web pages. It gives an illusion of movement and can be used to convey a sense of excitement or energy. In this article, we will show you how to create a wave animation in Flutter.
The animation is interesting to use in a Flutter app. It’s the foundation for a liquid motion.
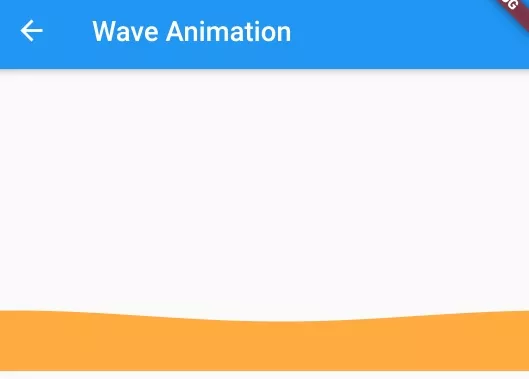
WaveContainer( width: double.infinity, height: 50, waveColor: Colors.orangeAccent)
class WaveContainer extends StatefulWidget { final Duration duration; final double height; final double width; final Color waveColor; const WaveContainer({ Key key, this.duration, @required this.height, @required this.width, this.waveColor, }) : super(key: key); @override _WaveContainerState createState() => _WaveContainerState(); } class _WaveContainerState extends State<WaveContainer> with TickerProviderStateMixin { AnimationController _animationController; Duration _duration; Color _waveColor; @override void initState() { super.initState(); _duration = widget.duration ?? const Duration(milliseconds: 1000); _animationController = AnimationController(vsync: this, duration: _duration); _waveColor = widget.waveColor ?? Colors.lightBlueAccent; _animationController.repeat(); } @override Widget build(BuildContext context) { return Container( width: widget.width, height: widget.height, child: AnimatedBuilder( animation: _animationController, builder: (BuildContext context, Widget child) { return CustomPaint( painter: WavePainter( waveAnimation: _animationController, waveColor: _waveColor), ); }, ), ); } @override void dispose() { _animationController?.stop(); _animationController?.dispose(); super.dispose(); } } class WavePainter extends CustomPainter { Animation<double> waveAnimation; Color waveColor; WavePainter({this.waveAnimation, this.waveColor}); @override void paint(Canvas canvas, Size size) { Path path = Path(); for (double i = 0.0; i < size.width; i++) { path.lineTo(i, sin((i / size.width * 2 * pi) + (waveAnimation.value * 2 * pi)) * 4); } path.lineTo(size.width, size.height); path.lineTo(0.0, size.height); path.close(); Paint wavePaint = Paint()..color = waveColor; canvas.drawPath(path, wavePaint); } @override bool shouldRepaint(CustomPainter oldDelegate) { return true; } }