How to Create a Glassmorphism Card in Flutter
Morphing design is a type of design that is transparent and dynamic. It relies on flowing lines and shapes to create a cohesive look that matches the movement and feel of the environment it is used in.
Glassmorphism is a great way to add some extra flair and creativity to your designs. It can take any background, whether it’s colorful or dull in coloration – like transparent glass!
With this style you get the ability not only to see through what lies beneath but also feel as if there are layers upon layer separated by thin sheets of material that have been carefully sculpted so they don’t hinder movement while still being obvious enough when viewed up close without ever becoming overwhelming on first glance due to their complexity.
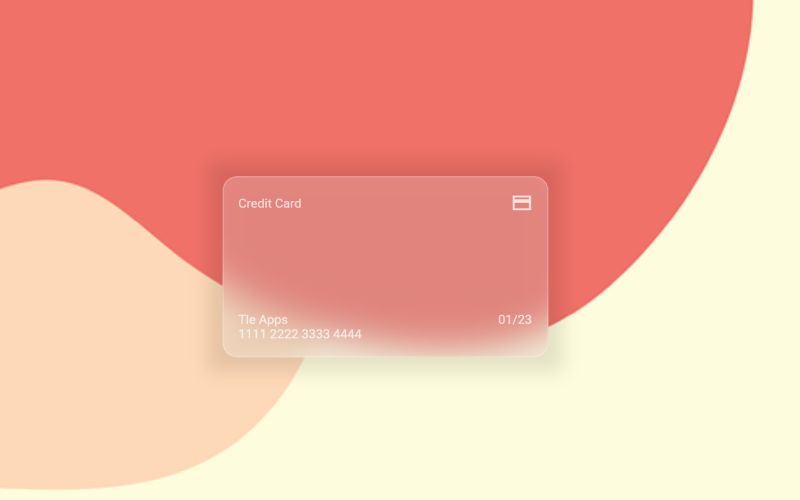
import 'dart:ui'; import 'package:flutter/material.dart'; class Homepage extends StatefulWidget { const Homepage({Key? key}) : super(key: key); @override _HomepageState createState() => _HomepageState(); } class _HomepageState extends State<Homepage> { @override Widget build(BuildContext context) { return Scaffold( body: Container( decoration: const BoxDecoration( image: const DecorationImage( image: const NetworkImage('https://cdn.pixabay.com/photo/2021/06/24/11/18/background-6360861_960_720.png'), fit: BoxFit.cover, ), ), child: Center( child: Container( decoration: BoxDecoration(boxShadow: [ BoxShadow( blurRadius: 16, spreadRadius: 16, color: Colors.black.withOpacity(0.1), ) ]), child: ClipRRect( borderRadius: BorderRadius.circular(16.0), child: BackdropFilter( filter: ImageFilter.blur( sigmaX: 20.0, sigmaY: 20.0, ), child: Container( width: 360, height: 200, decoration: BoxDecoration( color: Colors.white.withOpacity(0.2), borderRadius: BorderRadius.circular(16.0), border: Border.all( width: 1.5, color: Colors.white.withOpacity(0.2), )), child: Padding( padding: const EdgeInsets.all(16.0), child: Column( children: [ Row( mainAxisAlignment: MainAxisAlignment.spaceBetween, children: [ Text('Credit Card', style: TextStyle(color: Colors.white.withOpacity(0.75))), Icon(Icons.credit_card_sharp, color: Colors.white.withOpacity(0.75),) ], ), const Spacer(), Row( mainAxisAlignment: MainAxisAlignment.spaceBetween, children: [ Text('Tle Apps', style: TextStyle(color: Colors.white.withOpacity(0.75))), Text('01/23', style: TextStyle(color: Colors.white.withOpacity(0.75))), ], ), Row( mainAxisAlignment: MainAxisAlignment.spaceBetween, children: [ Text('1111 2222 3333 4444', style: TextStyle(color: Colors.white.withOpacity(0.75))), ], ) ], ), ) ), ), ), ), ), ), ); } }
Here is snippet of the container for reuse:
class GlassContainer extends StatelessWidget { final double? height; final double? width; final Widget? child; const GlassContainer({Key? key, this.child, this.height, this.width}) : super(key: key); @override Widget build(BuildContext context) { return Container( decoration: BoxDecoration(boxShadow: [ BoxShadow( blurRadius: 8, spreadRadius: 8, color: Colors.black.withOpacity(0.05), ) ]), child: ClipRRect( borderRadius: BorderRadius.circular(16.0), child: BackdropFilter( filter: ImageFilter.blur( sigmaX: 20.0, sigmaY: 20.0, ), child: Container( width: width ?? 360, height: height ?? 200, decoration: BoxDecoration( color: Colors.white.withOpacity(0.2), borderRadius: BorderRadius.circular(16.0), border: Border.all( width: 1.5, color: Colors.white.withOpacity(0.2), )), child: child!, ), ), ), ); } }