Display Counter Badge above an Icon in Flutter
If you’re a Flutter developer, you’re probably familiar with a counter badge. It’s a great way to show a count of something without having to use up valuable space on your screen. But what if you want to show the counter badge above an icon? In this post, we’ll show you how to do just that!
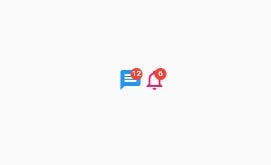
class MyApp extends StatelessWidget { @override Widget build(BuildContext context) { return MaterialApp( debugShowCheckedModeBanner: false, home: Scaffold( body: Center( child: Row( mainAxisAlignment: MainAxisAlignment.center, crossAxisAlignment: CrossAxisAlignment.center, children: const [ CounterIcon(icon: Icon(Icons.message, color: Colors.blue, size: 36), counter: 12), CounterIcon(icon: Icon(Icons.notifications_none_outlined, color: Colors.pink, size: 36), counter: 6), ], ), ), ), ); } } class CounterIcon extends StatelessWidget { final Icon? icon; final int? counter; const CounterIcon({Key? key, this.icon, this.counter}) : super(key: key); @override Widget build(BuildContext context) { return Stack(children: <Widget>[ icon!, Positioned( right: 0, child: Container( padding: const EdgeInsets.all(2), decoration: BoxDecoration( color: Colors.red, borderRadius: BorderRadius.circular(6), ), constraints: const BoxConstraints( minWidth: 16, minHeight: 16, ), child: Text( '$counter', style: const TextStyle( color: Colors.white, fontSize: 10, ), textAlign: TextAlign.center, ), ), ) ]); } }