Create a Wave-shaped AppBar in Flutter
The AppBar class is the primary widget for displaying actions and navigation controls in a Flutter app. It can be either a standalone widget or a child of a Scaffold.
A wave-shaped AppBar can be created in Flutter using the ClipPath widget. To create a wave AppBar, we can use the CustomPaint widget and pass it to a CustomPainter that draws a curved shape.
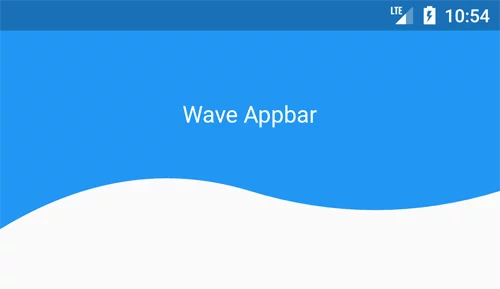
class MainAppBar extends StatelessWidget implements PreferredSizeWidget { final Text title; final double barHeight = 50.0; MainAppBar({Key key, this.title}) : super(key: key); @override Size get preferredSize => Size.fromHeight(kToolbarHeight + 100.0); @override Widget build(BuildContext context) { return PreferredSize( child: ClipPath( clipper: WaveClip(), child: Container( color: Colors.blue, child: Column( mainAxisAlignment: MainAxisAlignment.center, children: <Widget>[ title, ], ), ), ), preferredSize: Size.fromHeight(kToolbarHeight + 100)); } } class WaveClip extends CustomClipper<Path> { @override Path getClip(Size size) { Path path = new Path(); final lowPoint = size.height - 30; final highPoint = size.height - 60; path.lineTo(0, size.height); path.quadraticBezierTo( size.width / 4, highPoint, size.width / 2, lowPoint); path.quadraticBezierTo( 3 / 4 * size.width, size.height, size.width, lowPoint); path.lineTo(size.width, 0); return path; } @override bool shouldReclip(CustomClipper<Path> oldClipper) { return false; } }