Add Padding to Container Widget in Flutter
Padding is the space inside the container and between the child widget’s edge and the container’s edge.
In Flutter, we can set padding for a widget by giving value to the padding property with an EdgeInsets class object. The EdgeInsets class has a top, right, bottom, and left properties to set padding for each side separately.
Another method is to wrap the Container widget with the Padding widget. The Padding widget also has a padding property that takes in an EdgeInsets class object.
By default, the padding is 0.0 pixels. But we can set it to any value we want, and it is common to set it to 8.0 or 16.0 (the number of pixels on most devices).
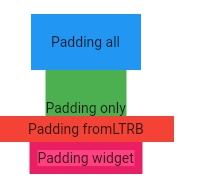
import 'package:flutter/material.dart'; void main() { runApp(MyApp()); } class MyApp extends StatelessWidget { String? productName = "Crystal Ring"; @override Widget build(BuildContext context) { return MaterialApp( debugShowCheckedModeBanner: false, home: Scaffold( backgroundColor: Colors.white, body: Column( mainAxisAlignment: MainAxisAlignment.center, crossAxisAlignment: CrossAxisAlignment.center, children: [ Container( padding: const EdgeInsets.all(20), child: const Text("Padding all"), color: Colors.blue), Container( padding: const EdgeInsets.only(top: 30), child: const Text("Padding only"), color: Colors.green), Container( padding: const EdgeInsets.fromLTRB(30, 5, 30, 5), child: const Text("Padding fromLTRB"), color: Colors.red), Container( color: Colors.pink, //added Container outside to show background color child: Padding( padding: const EdgeInsets.all(8.0), child: Container( child: const Text("Padding widget"), color: Colors.pinkAccent), ), ), ]), ), ); } }