Django Boilerplates
Django is a free and open-source web application framework, written in Python. It follows the model-view-template (MVT) architectural pattern. This article will list some of the best Django boilerplates to help you get started with your next project.
Best Django Boilerplates
Cookiecutter Django
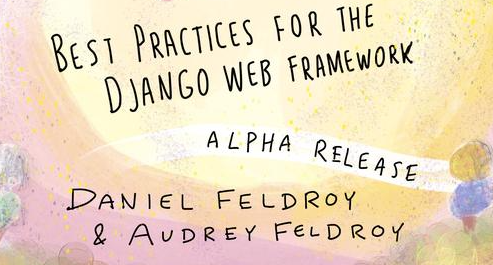
If you’re looking for a quick and easy way to get your Django project up and running, Cookiecutter Django is the answer. This open-source framework provides everything you need to start with 100% test coverage, including Twitter Bootstrap v5, 12-Factor settings via django-environ, and optimized development and production settings.
You can also easily register new users with django-allauth, store your media files with Amazon S3 or Google Cloud Storage, send emails via Anymail (Mailgun by default or Amazon SES if AWS is sa elected cloud provider), and run tests with unittest or pytest.
Plus, its customizable PostgreSQL version allows you to choose the database that best suits your needs.
A Minimalistic Modern Django Boilerplate
Django is a high-level Python web framework that encourages rapid development and clean, pragmatic design. Boilerplates are templates for starting or scaffolding a project quickly. This Django Boilerplate is a minimalistic, modern template meant to be cloned as a starter code for future tutorials.
It has been fully featured with the goal of being extensible so that you can add your own preferences and customize it with more complicated options.
The idea is that once you build and understand the basic example, you could swab parts of it with your own preferences or simply build on top of this one. This boilerplate replaces the default startproject Django code.
django-boilerplate
When starting a new Django project, it can be helpful to use a boilerplate as a foundation. This will give you a basic structure to work with and help avoid common problems.
The django-boilerplate is one such boilerplate, and it includes everything you need to get started with a simple Django app. It comes with pre-defined settings, templates, and a CRUD (Create, Read, Update, Delete) module. You can also use the project as a base for more complex apps.
django-code-generator
Django-code-generator helps developers speed up the process of creating a Django project. It does this by automatically generating code from your models, allowing you to skip the time-consuming and often error-prone task of creating all the necessary files by hand.
This project also includes templates for generating an API or admin for your app. If you need to create code for something specific that isn’t covered by the provided templates, don’t worry – you can create your own and share them with others.
Django Features
Static File Serving
Django doesn’t serve static files by default; for this, you would need to set up some kind of HTTP server like Apache or Nginx. If you’re developing locally, however, Django provides a way to serve static files itself.
Virtual Environment
Django is not installed system-wide. It creates its own virtual environment in the project folder which contains its own installation directories. The easy_install package manager currently installs projects in the current directory for this reason, but PyPI recommends installing packages in site packages.
Database Migrations
Django applications can be configured to automatically collect changes to models (known as “migrations”). When these migrations are applied, the database schemas are updated to match the current set of models. Django includes a utility that allows you to manage your database schemas via the command line.
Management Commands
Django comes with a management command that lets you interact with the database; for example creating and dropping databases, inspecting the current database state, running raw SQL commands, and restoring from an incremental backup archive.
You can create your own management commands too; several third-party Django applications (e.g., Sphinx, Pinax) provide a range of management commands.
Template System
Django’s template system is very powerful and flexible but can be tricky to get started with. It uses a curly brace syntax which mustn’t be typed in literally. If you want to escape the braces within your strings, you’ll have to backslash them, which can result in some pretty confusing errors.
ORM
Django’s ORM has a reputation for being difficult to work with due to its implementation details and lack of support for advanced features such as joins. It is generally built around the idea of denormalizing your data from your relational database into your models in order to reduce the number of queries that must be made.
Template Tags/Filters
Although there are several good template libraries available for Python (e.g., Jinja2), Django’s template tags and filters are very fast because they’re written in C as a Python extension, Cython, or PyPy. Both render out templates before firing up the main Python interpreter, so the output is a lot quicker.
Block System & Multiple Inheritance
Django uses a block system to separate out different sections of templates and avoid name clashes between blocks with the same name in different templates. It also implements multiple inheritances which can be useful for sharing common sections of code across several models without having to create a common base model.
Permissions and Authorization
Django allows permissions (with regards to object-level or database-level access) to be defined in models and checked at the point of accessing objects. It also provides an optional user authentication that can be linked to these permissions; if a user doesn’t have permission to perform an action, the application won’t let them do it.
Caching
Django has extensive caching support which can be used at the object level (i.e., cache an individual model instance) or globally (i.e., set a global default for all views). This makes it great for improving performance. There are also several pluggable cache backends available.