How to Create and Use Enum in Dart?
Enums are one of the most important data types in any programming language. They allow you to create a custom data type that is restricted to a specific set of values. In Dart, enums are created using the enum keyword. In this tutorial, we will show you how to create an enum and use it in your code.
Define an enum
//syntax enum enum_name { enumeration list } //example enum Direction { north, south, east, west }
Use enum
var direction = Direction.south; switch (direction) { case Direction.north: print("You are going North!"); break; case Direction.south: print("You are going South!"); break; case Direction.east: print("You are going East!"); break; case Direction.west: print("You are going West!"); break; }
Loop an enum
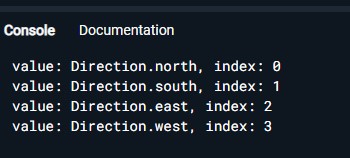
for (var v in Direction.values) { print('value: $v, index: ${v.index}'); }
Get values with extension
enum QtyStatus { none, add, change, remove } extension QtyStatusExtension on QtyStatus { String get status { switch (this) { case QtyStatus.add: return 'ADD'; case QtyStatus.change: return 'CHANGE'; case QtyStatus.remove: return 'REMOVE'; default: return 'NONE'; } }