The Best PHP Libraries to Work with Microsoft Word in 2023
You know Microsoft Word is an amazing software program. However, it can be a bit lacking when it comes to making a PHP app that depends on reading and writing to this format.
Luckily there are PHP libraries out there that will help make your life easier by doing things like changing the font size or adding borders around paragraph sections in MS Word. With these libraries, you can focus on what you’re good at and let them take care of the formatting for you.
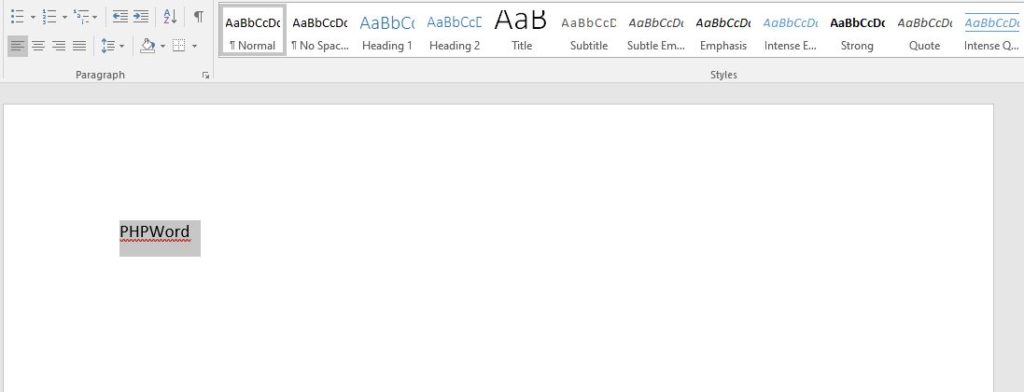
PHPWord
Programming that can write office documents is not something to take lightly. Creating a word processor from scratch would be nearly impossible and a monstrosity like Microsoft Word has hundreds of thousands of lines of code just for the “core” program!
Why waste your time when you don’t have to? With PHPWord, there’s never been an easier or more reliable way to create, edit, and output office documents from your PHP applications.
PHPWord supports Microsoft Office Open XML, OpenDocument or ODF, Rich Text Format, HTML, and PDF.
The PHPWord library allows organizations to create Office documents dynamically with the power of PHP. It will allow marketers and developers alike to create compositions that range from templates and letters to presentation decks, such as PowerPoint presentations.
Site owners can take advantage of the facility in which they can use XSL 1.0 style sheets with PHPWord’s OOXML template support to create dynamic headers, main document parts, and footers.
Furthermore, the library contains features that make it easy to work with tables. For example, you can set a table’s properties such as width, height, and a number of columns so that it fits the size of the page perfectly.
require_once 'bootstrap.php'; // Creating the new document... $phpWord = new \PhpOffice\PhpWord\PhpWord(); /* Note: any element you append to a document must reside inside of a Section. */ // Adding an empty Section to the document... $section = $phpWord->addSection(); // Adding Text element to the Section having font styled by default... $section->addText( '"Learn from yesterday, live for today, hope for tomorrow. ' . 'The important thing is not to stop questioning." ' . '(Albert Einstein)' ); /* * Note: it's possible to customize font style of the Text element you add in three ways: * - inline; * - using named font style (new font style object will be implicitly created); * - using explicitly created font style object. */ // Adding Text element with font customized inline... $section->addText( '"Great achievement is usually born of great sacrifice, ' . 'and is never the result of selfishness." ' . '(Napoleon Hill)', array('name' => 'Tahoma', 'size' => 10) ); // Adding Text element with font customized using named font style... $fontStyleName = 'oneUserDefinedStyle'; $phpWord->addFontStyle( $fontStyleName, array('name' => 'Tahoma', 'size' => 10, 'color' => '1B2232', 'bold' => true) ); $section->addText( '"The greatest accomplishment is not in never falling, ' . 'but in rising again after you fall." ' . '(Vince Lombardi)', $fontStyleName ); // Adding Text element with font customized using explicitly created font style object... $fontStyle = new \PhpOffice\PhpWord\Style\Font(); $fontStyle->setBold(true); $fontStyle->setName('Tahoma'); $fontStyle->setSize(13); $myTextElement = $section->addText('"Believe you can and you\'re halfway there." (Theodor Roosevelt)'); $myTextElement->setFontStyle($fontStyle); // Saving the document as OOXML file... $objWriter = \PhpOffice\PhpWord\IOFactory::createWriter($phpWord, 'Word2007'); $objWriter->save('helloWorld.docx'); // Saving the document as ODF file... $objWriter = \PhpOffice\PhpWord\IOFactory::createWriter($phpWord, 'ODText'); $objWriter->save('helloWorld.odt'); // Saving the document as HTML file... $objWriter = \PhpOffice\PhpWord\IOFactory::createWriter($phpWord, 'HTML'); $objWriter->save('helloWorld.html');
You can also insert images into tables and have them scale to the size of the table. The library also includes features for inserting hyperlinks, lists, and form fields into documents.
Overall, the PHPWord library is an impressive tool that will allow developers to create dynamic Office documents with PHP. It should be noted that this library requires PHP 5.3.3 or higher in order to function.