6 Best PHP Libraries to Read Barcodes and QR Codes
A barcode is an optical machine-readable representation of data relating to the object to which it is attached. Barcodes are widely used in inventory control, supermarket check-out systems, and many other applications.
If you’ve ever had to deal with barcodes, you know that they can be a bit of a pain. There are all sorts of different formats, and keeping track of them all can be a real headache. Fortunately, many PHP libraries can help make reading and generating barcodes a lot easier.
tcpdf
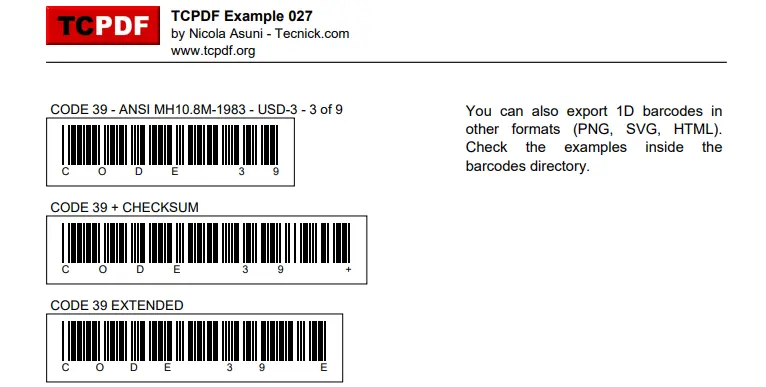
If you need to generate PDF documents or barcodes in PHP, TCPDF is the class for you. It supports 1D and 2D barcodes, including CODE 39, ANSI MH10.8M-1983, USD-3, 3 of 9, CODE 93, USS-93, EAN 8, EAN 13, UPC-A, QR-Code, and many more.
In other words, it can handle just about any barcode you’re likely to need. And if you need to generate PDFs as well as barcodes, TCPDF has you covered there too – it’s a full-featured PDF generation library.
public function getBarcodeSVG($w=3, $h=3, $color='black') { // send headers $code = $this->getBarcodeSVGcode($w, $h, $color); header('Content-Type: application/svg+xml'); header('Cache-Control: public, must-revalidate, max-age=0'); // HTTP/1.1 header('Pragma: public'); header('Expires: Sat, 26 Jul 1997 05:00:00 GMT'); // Date in the past header('Last-Modified: '.gmdate('D, d M Y H:i:s').' GMT'); header('Content-Disposition: inline; filename="'.md5($code).'.svg";'); //header('Content-Length: '.strlen($code)); echo $code; }
YellowskiesQRcodeBundle
The YellowskiesQRcodeBundle is a Symfony4/5/6 Barcode Generator Bundle that makes it easy to generate barcodes in your Symfony project. This bundle makes it simple to generate both 2D and 1D barcodes in three different output formats: HTML, PNG, and SVG canvas.
Plus, thanks to the Twig integration, you can just use an extension function in your template to generate the barcode. And if you need more power or flexibility, the bundle is built on top of the tc-lib-barcode project, so you’ve got access to all its features.
$options = array( 'code' => 'string to encode', 'type' => 'c128', 'format' => 'html', ); $barcode = $this->get('skies_barcode.generator')->generate($options); return new Response($barcode);
php-barcode-generator
If you need to generate barcodes in PHP, this easy-to-use barcode generator is a great option. It supports the most popular 1D barcode standards and can create PNG, JPG, SVG, or HTML images.
The codebase is based on the TCPDF barcode generator by Nicola Asuni, so it’s licensed under LGPLv3. If you want to generate PNG or JPG images, you’ll need the GD library or Imagick installed.
// This will output the barcode as HTML output to display in the browser $generator = new Picqer\Barcode\BarcodeGeneratorHTML(); echo $generator->getBarcode('081231723897', $generator::TYPE_CODE_128); $generatorSVG = new Picqer\Barcode\BarcodeGeneratorSVG(); // Vector based SVG $generatorPNG = new Picqer\Barcode\BarcodeGeneratorPNG(); // Pixel based PNG $generatorJPG = new Picqer\Barcode\BarcodeGeneratorJPG(); // Pixel based JPG $generatorHTML = new Picqer\Barcode\BarcodeGeneratorHTML(); // Pixel based HTML $generatorHTML = new Picqer\Barcode\BarcodeGeneratorDynamicHTML(); // Vector based HTML
Unfortunately, there is no support for any 2D barcodes like QR codes.
php-barcodes
php-barcodes is a class that can check EAN8, EAN13, UPC, and GTIN barcodes to see if they’re valid. It does this by using the check digit, which is the last digit in the barcode. If the check digit doesn’t match up, then the barcode is invalid.
This class can be really helpful if you need to deal with a lot of barcodes on a regular basis. It can save you a lot of time and hassle, and make sure that you always have valid barcodes. So if you’re looking for an easier way to deal with barcodes, php-barcodes are definitely worth checking out.
// Class instantation $barcode = '5060411950139'; $bc_validator = new \violuke\Barcodes\BarcodeValidator($barcode); // Check barcode is in valid format if ($bc_validator->isValid()) { echo 'Valid :)'; } else { echo 'Invalid :('; } // Get the barcode type echo 'Barcode is in format of ' . $bc_validator->getType(); // Possible formats returned are: // (string) "GTIN" which equals constant \violuke\Barcodes\BarcodeValidator::TYPE_GTIN // (string) "EAN-8" which equals constant \violuke\Barcodes\BarcodeValidator::TYPE_EAN_8 // (string) "EAN" which equals constant \violuke\Barcodes\BarcodeValidator::TYPE_EAN // (string) "EAN Restricted" which equals constant \violuke\Barcodes\BarcodeValidator::TYPE_EAN_RESTRICTED // (string) "UPC" which equals constant \violuke\Barcodes\BarcodeValidator::TYPE_UPC // (string) "UPC Coupon Code" which equals constant \violuke\Barcodes\BarcodeValidator::TYPE_UPC_COUPON_CODE // Returns the barcode in GTIN-14 format $bc_validator->getGTIN14() // Returns the barcode as entered $bc_validator->getBarcode()
zebra
Zebra is a PHP library for easily creating and sending ZPL (Zebra Programming Language) commands to Zebra label printers over a network connection. It can also be used to convert images into ASCII hex code that can be included in your ZPL code for printing.
The library is easy to use and easy to read, making it a great option for anyone who needs to quickly create and send ZPL commands to a Zebra label printer.
use Zebra\Client; use Zebra\Zpl\Image; use Zebra\Zpl\Builder; use Zebra\Zpl\GdDecoder; $decoder = GdDecoder::fromPath('example.png'); $image = new Image($decoder); $zpl = new Builder(); $zpl->fo(50, 50)->gf($image)->fs(); $client = new Client('10.0.0.50'); $client->send($zpl);
barcode
In Laravel, there is a package called barcode that helps with generating barcodes. This package makes it easy to generate barcodes in Laravel by using TCPDF, an open-source PHP class for creating PDF documents. With this package, you can easily create and display barcodes in your Laravel applications.
echo DNS1D::getBarcodeSVG('4445645656', 'PHARMA2T'); echo DNS1D::getBarcodeHTML('4445645656', 'PHARMA2T'); echo '<img src="data:image/png,' . DNS1D::getBarcodePNG('4', 'C39+') . '" alt="barcode" />'; echo DNS1D::getBarcodePNGPath('4445645656', 'PHARMA2T'); echo '<img src="data:image/png;base64,' . DNS1D::getBarcodePNG('4', 'C39+') . '" alt="barcode" />';