5 Best Flutter Packages for ML and AI in 2023
Machine learning (ML) and artificial intelligence (AI) are two of the most talked about topics in tech right now. And for good reason – they have the potential to change the world as we know it. But what are they, exactly? And more importantly, how can you use them in your own projects?
In this article, we’ll take a closer look at some of the best packages available for Flutter ML and AI development.
Best Flutter Packages for Machine Learning and AI
tflite_flutter
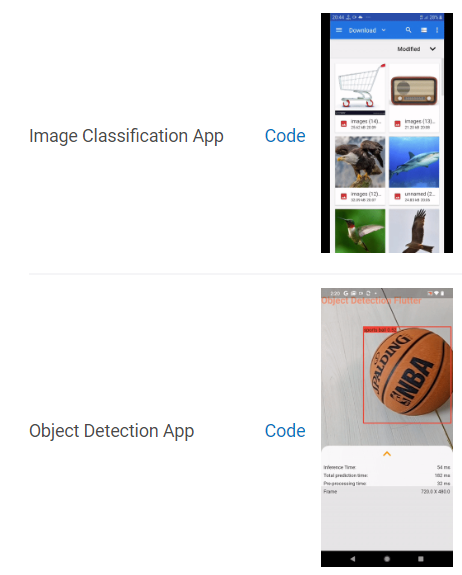
TensorFlow Lite is an open-source deep learning framework for on-device inference. It is developed by the Google Brain team. This platform has been growing in popularity because it offers a fast and flexible solution for deploying models on mobile and embedded devices.
The TensorFlow Lite Flutter package enables you to use any TensorFlow Lite model in your Flutter apps by providing a Dart API for accessing TensorFlow Lite’s Interpreter API. This package also supports acceleration using multi-threading and delegate support, similar to the TensorFlow Lite Java API. Inference speeds are also close to native Android apps built using the Java API.
//Android GpuDelegateV2 final gpuDelegateV2 = GpuDelegateV2( options: GpuDelegateOptionsV2( false, TfLiteGpuInferenceUsage.fastSingleAnswer, TfLiteGpuInferencePriority.minLatency, TfLiteGpuInferencePriority.auto, TfLiteGpuInferencePriority.auto, )); var interpreterOptions = InterpreterOptions()..addDelegate(gpuDelegateV2); final interpreter = await Interpreter.fromAsset('your_model.tflite', options: interpreterOptions);
You can choose to use any TensorFlow version by building binaries locally. You can run inference in different isolates to prevent jank in the UI thread.
In addition, the TensorFlow Lite Flutter plugin offers acceleration support using NNAPI, GPU delegates on Android, Metal and CoreML delegates on iOS, and XNNPack delegates on Desktop platforms.
flutter_vision
If you’re looking for a Flutter plugin that supports both Yolov5 and Tesseract v4 with TensorFlow Lite 2.x, you should try flutter_vision. This plugin offers support for object detection and OCR on both iOS and Android, making it a great choice for those who need to support multiple platforms.
//load model final responseHandler = await vision.loadOcrModel( labels: 'assets/labels.txt', modelPath: 'assets/yolov5.tflite', args: { 'psm': '11', 'oem': '1', 'preserve_interword_spaces': '1', }, language: 'spa', numThreads: 1, useGpu: false);
In addition, flutter_vision offers a variety of other features that make it an essential tool for those working with Flutter and TensorFlow Lite.
ml_algo
The ml_algo library is mainly for people who are interested in both the Dart language and data science. The purpose of the library is to provide native Dart implementations of machine learning algorithms. The library is for use on the Dart VM and Flutter, and cannot be used in web applications.
import 'dart:io'; void main() { // ... final fileName = 'diabetes_classifier.json'; final file = File(fileName); final encodedModel = await file.readAsString(); final classifier = LogisticRegressor.fromJson(encodedModel); final unlabelledData = await fromCsv('some_unlabelled_data.csv'); final prediction = classifier.predict(unlabelledData); print(prediction.header); // ('class variable (0 or 1)') print(prediction.rows);
The ml_algo library aims to provide an easy-to-use API, with good documentation and support for common tasks such as pre-processing data, training models, and making predictions. The library also includes a number of popular machine learning algorithms, such as decision trees, KDtree-based data retrieval, and more.
learning
With the learning package, it’s easy to get started with machine learning in Flutter. The Machine Learning Kit consists of 13 different sub-packages, each of which can be added separately based on your needs.
import 'package:flutter/material.dart'; import 'package:learning_input_image/learning_input_image.dart'; import 'package:learning_text_recognition/learning_text_recognition.dart'; import 'package:provider/provider.dart'; void main() { runApp(MyApp()); } class MyApp extends StatelessWidget { @override Widget build(BuildContext context) { return MaterialApp( debugShowCheckedModeBanner: false, theme: ThemeData( primarySwatch: Colors.lightBlue, visualDensity: VisualDensity.adaptivePlatformDensity, primaryTextTheme: TextTheme( headline6: TextStyle(color: Colors.white), ), ), home: ChangeNotifierProvider( create: (_) => TextRecognitionState(), child: TextRecognitionPage(), ), ); } } class TextRecognitionPage extends StatefulWidget { @override _TextRecognitionPageState createState() => _TextRecognitionPageState(); } class _TextRecognitionPageState extends State<TextRecognitionPage> { TextRecognition? _textRecognition = TextRecognition(); /* TextRecognition? _textRecognition = TextRecognition( options: TextRecognitionOptions.Japanese ); */ @override void dispose() { _textRecognition?.dispose(); super.dispose(); } Future<void> _startRecognition(InputImage image) async { TextRecognitionState state = Provider.of(context, listen: false); if (state.isNotProcessing) { state.startProcessing(); state.image = image; state.data = await _textRecognition?.process(image); state.stopProcessing(); } } @override Widget build(BuildContext context) { return InputCameraView( mode: InputCameraMode.gallery, // resolutionPreset: ResolutionPreset.high, title: 'Text Recognition', onImage: _startRecognition, overlay: Consumer<TextRecognitionState>( builder: (_, state, __) { if (state.isNotEmpty) { return Center( child: Container( padding: EdgeInsets.symmetric(vertical: 10, horizontal: 16), decoration: BoxDecoration( color: Colors.white.withOpacity(0.8), borderRadius: BorderRadius.all(Radius.circular(4.0)), ), child: Text( state.text, style: TextStyle( fontWeight: FontWeight.w500, ), ), ), ); } return Container(); }, ), ); } } class TextRecognitionState extends ChangeNotifier { InputImage? _image; RecognizedText? _data; bool _isProcessing = false; InputImage? get image => _image; RecognizedText? get data => _data; String get text => _data!.text; bool get isNotProcessing => !_isProcessing; bool get isNotEmpty => _data != null && text.isNotEmpty; void startProcessing() { _isProcessing = true; notifyListeners(); } void stopProcessing() { _isProcessing = false; notifyListeners(); } set image(InputImage? image) { _image = image; notifyListeners(); } set data(RecognizedText? data) { _data = data; notifyListeners(); } }
Each package focuses on a specific task, such as natural language processing, face recognition, image labeling, or optical character recognition. By adding only the packages you need, you can keep your machine learning program simple and efficient.
dart_ml
The dart_ml package is a great way to add machine learning functionality to your apps. It includes a variety of algorithms that can be used for classification, regression, and other tasks.
var dataset = [ [2.7810836, 2.550537003, 0], [1.465489372, 2.362125076, 0], [3.396561688, 4.400293529, 0], [1.38807019, 1.850220317, 0], [3.06407232, 3.005305973, 0], [7.627531214, 2.759262235, 1], [5.332441248, 2.088626775, 1], [6.922596716, 1.77106367, 1], [8.675418651, -0.242068655, 1], [7.673756466, 3.508563011, 1] ]; var predicted = logreg(dataset, dataset[0], 0.3, 100)); // (train, test, l_rate, n_epoch) print(predicted); //0, returns the predicted class
The k-nearest neighbor’s algorithm is particularly useful for classification problems, while the logistic regressor is a good choice for regression tasks. With dart_ml, you can easily add machine learning to your apps without having to worry about the details of the algorithms.